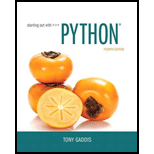
Pet Class
The Pet class
Write a class named Pet, which should have the following data attributes:
■ _ _ name (for the name of a pet)
■ _ _ animal_type (for the type of animal that a pet is. Example values are 'Dog', 'Cat', and 'Bird’)
■ _ _ age (for the pet’s age)
The Pet class should have an _ _init_ _ method that creates these attributes. It should also have the following methods:
■ set_name
This method assigns a value to the _ _name field.
■ set_animal_type
This method assigns a value to the _ _animal_type field.
■ set_age
This method assigns a value to the _ _age field.
■ get_name
This method returns the value of the _ _name field.
■ get_animal_type
This method returns the value of the _ _animal_type field.
■ get_age
This method returns the value of the _ _age field.
Once you have written the class, write a
stored as the object’s attributes. Use the object's accessor methods to retrieve the pet’s name, type, and age and display this data on the screen.

Learn your wayIncludes step-by-step video

Chapter 10 Solutions
Starting Out with Python (4th Edition)
Additional Engineering Textbook Solutions
Introduction To Programming Using Visual Basic (11th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Programming in C
Computer Systems: A Programmer's Perspective (3rd Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Problem Solving with C++ (10th Edition)
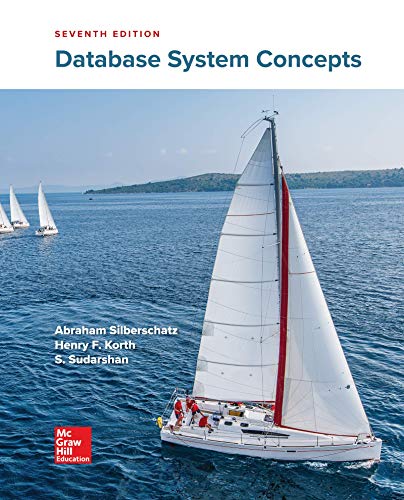
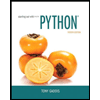
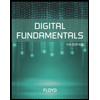
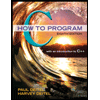
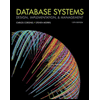
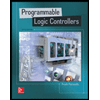
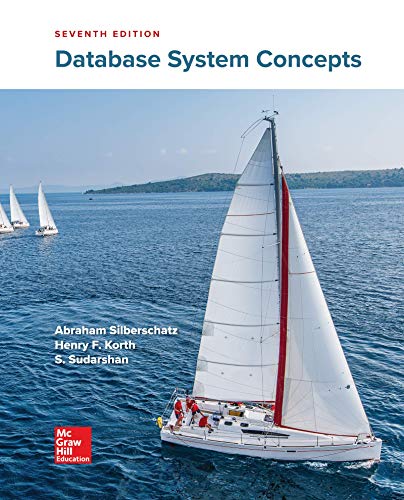
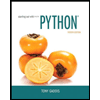
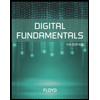
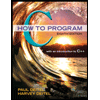
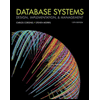
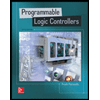