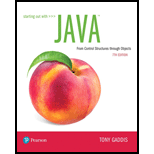
Concept explainers
SavingsAccount Class
Design a SavingsAccount class that stores a savings account’s annual interest rate and balance. The class constructor should accept the amount of the savings account’s starting balance. The class should also have methods for subtracting the amount of a withdrawal, adding the amount of a deposit, and adding the amount of monthly interest to the balance. The monthly interest rate is the annual interest rate divided by twelve. To add the monthly interest to the balance, multiply the monthly interest rate by the balance, and add the result to the balance.
Test the class in a
- a. Ask the user for the amount deposited into the account during the month. Use the class method to add this amount to the account balance.
- b. Ask the user for the amount withdrawn from the account during the month. Use the class method to subtract this amount from the account balance.
- c. Use the class method to calculate the monthly interest.
After the last iteration, the program should display the ending balance, the total amount of deposits, the total amount of withdrawals, and the total interest earned.

Learn your wayIncludes step-by-step video

Chapter 6 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Starting Out with Python (3rd Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Artificial Intelligence: A Modern Approach
Starting out with Visual C# (4th Edition)
Concepts of Programming Languages (11th Edition)
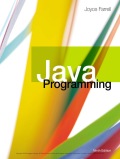
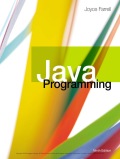